posted February 20, 2013
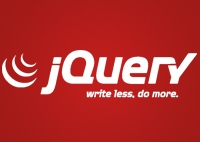
Recently, while working on a mobile version of a Website, I was asked to hide the search bar that's traditionally at the top of a web page, and replace it with a clickable button. When clicked, this button would in turn reveal the search bar. The idea behind this user interaction was to save valuable screen real estate by hiding the search bar, which is only occasionally used.
I used CSS (display:none) to hide the search bar initially, and to display the button that would reveal it.
Here's a little legend to explain what my elements are in the examples below:
- #search-box-trigger = the clickable button that will show the search box
- #search-box = the div containing the search form
- #searchText = the text input field in the search form
Next I used a little jQuery to make the search bar appear on click of the button:
$('#search-box-trigger').click(function () {
$('#search-box').toggle();
// toggle is a jquery function that detects the default
// display of an element and switches it to its opposite,
// so from NONE to BLOCK, or from BLOCK to NONE
$(this).toggle();
// THIS refers to the element you first call with your function,
// in this case #search-box-trigger
});
However, just hiding the button and showing the search box required the user to then click into the search box again. Since the user already showed intent at searching, by clicking the button, why not give the search bar "focus" (meaning, place the cursor inside the search bar) for the user, saving them an extra step, and causing their mobile device's keypad to come up so he can start entering their search term?
By adding this line, I give the search text input field focus:
$("#search-box #searchText").focus();
So the final, complete jquery snippet looks like this:
$('#search-box-trigger').click(function () {
$('#search-box').toggle();
$(this).toggle();
$("#search-box #searchText").focus();
});